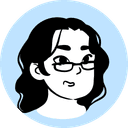
Understanding the Scope of JavaScript Variables
Scope
is literally translated as "range" or "area", and developers mainly use it to mean "the valid range of variables" or "scope of application".
While other programming languages use curly braces or indentation to separate code blocks and define a scope, how does JavaScript define its scope?
What is Scope?
In JavaScript
, the concept of scope defines the range in which a variable, function, or object can be accessed based on where it is declared.
Simply put, you can think of scope as the rules governing where a variable can be used within the code.
Types of Scope
In JavaScript
, the types of scope include global scope, function scope, and block scope.
1. Global Scope
Global scope allows variables or functions declared at the top level of the code to be accessed anywhere in the application.
const name = "Jang"; // global variable
function printName() {
console.log(name); // accessible here
}
if (true) {
console.log(name); // accessible here
}
console.log(name); // accessible here
In the above code, name
is a variable declared at the top level of the code, so it can be accessed both inside and outside of functions or if
statements.
2. Local Scope
Local scope refers to the range in which variables declared within a specific function or block can only be used in that local area.
function calculateSalary() {
const name = 'jangdu'; // local variable
const age = 8; // local variable
console.log(name, age); // accessible within the function
}
console.log(name, age); // ERROR! Cannot be accessed outside the function
In the example code, the two variables can be accessed within the declared function, but if accessed outside the function, an error occurs and the code cannot be executed.
3. Block Scope
Block scope is the range that is valid only within the curly braces {}
. Variables declared with let
and const
have block scope.
if (true) {
let department = "개발팀"; // accessible only within the block
const position = "프론트엔드"; // accessible only within the block
console.log(department); // "개발팀"
console.log(position); // "프론트엔드"
}
console.log(department); // Error! Cannot be accessed outside the block
console.log(position); // Error! Cannot be accessed outside the block
In the above code, department
and position
are variables declared within the if
statement, and can be accessed within the code block of the if
statement, where their values are printed correctly.
However, if you try to access the variables outside of the code block, an error occurs and the code will not execute.
Scope Chaining
Scope chaining describes the process of finding variables in JavaScript.
In the example code below, when the variable food
is declared three times and accessed, it requires a process to determine which variable is being referred to.
let food = "김치"; // global variable
function outerFunction() {
let food = "피자"; // local variable
function innerFunction() {
let food = "과자"; // local variable
console.log(food); // 과자
}
console.log(food); // 피자
innerFunction();
}
outerFunction();
JavaScript looks for the closest variable among accessible scopes, moving outward. Therefore, the food
used inside innerFunction
outputs the value of the closest variable, which is "과자", while in outerFunction
, it outputs "피자".
By understanding and utilizing the properties of scope well, you can avoid variable name collisions in your code, enhancing code stability. Additionally, debugging becomes easier when issues arise, and memory can be managed more efficiently.
In summary, scope is a fundamental concept in JavaScript that helps write cleaner and more maintainable code.